Webhooks
Binance uses webhooks to notify you when something happens. We will automatically send you a notification immediately to keep you up-to-date on your order status.
You will be able to configure webhook endpoints via the Merchant Management Platform.
#
Setting up WebhooksFor security purposes, Binance will add a signature for webhook notification. Merchants need to verify the signature using the public key issued from Binance Pay.
#
How it worksConfigure the webhook endpoints in the Merchant Management Platform (MMP).
Build the payload and verify the signature on your application. Please note that the certificate endpoint is fixed.
Identify the events you want to be notified of.
Get notified automatically on your order status.
After receiving the notification, please acknowledge it by returning a HTTP 200 and “SUCCESS” in the response body.
#
Webhook Configuration in MMPUnder the [Developer] tab, select [Webhooks] then [Edit], input your site URL to receive the webhook notification and description.
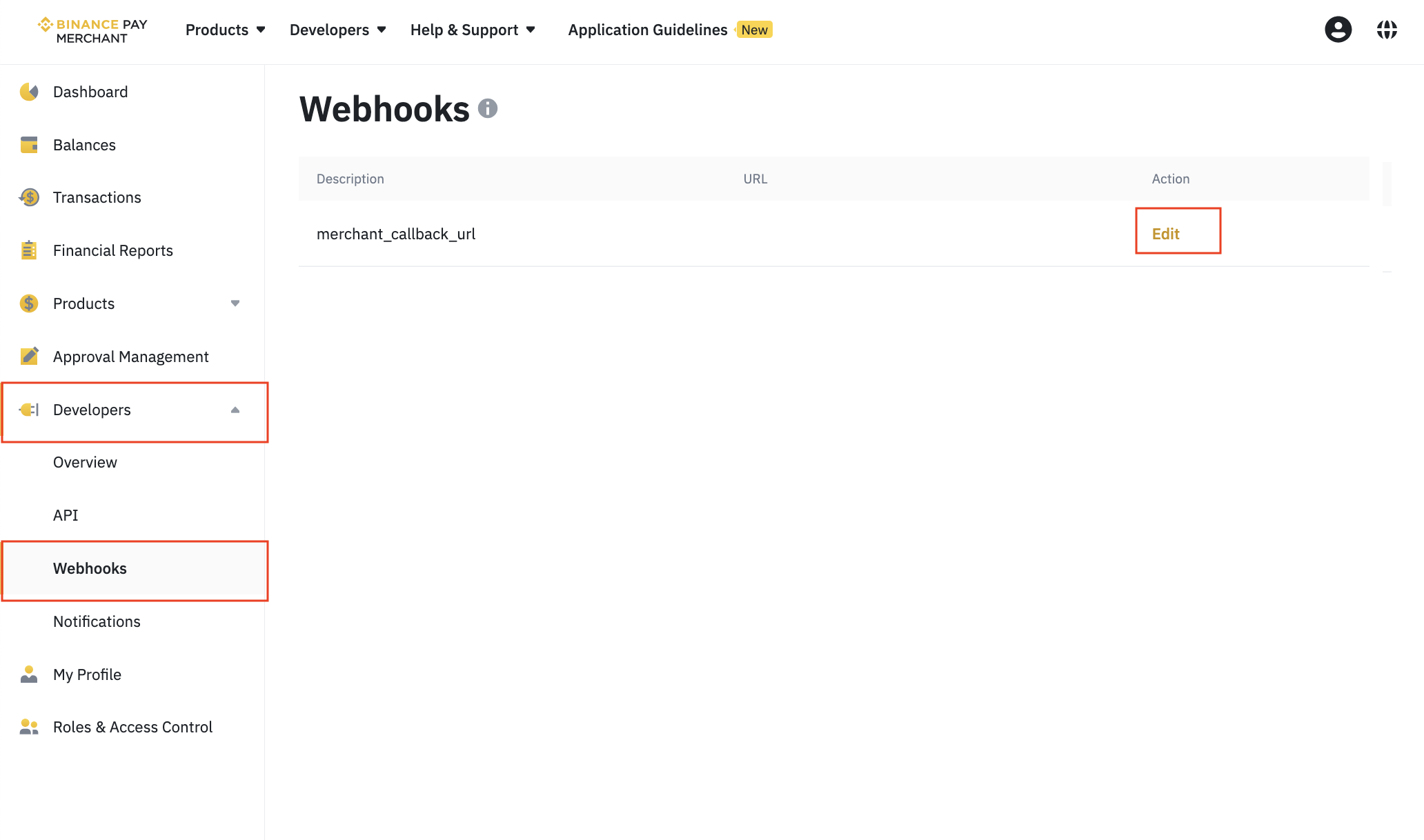
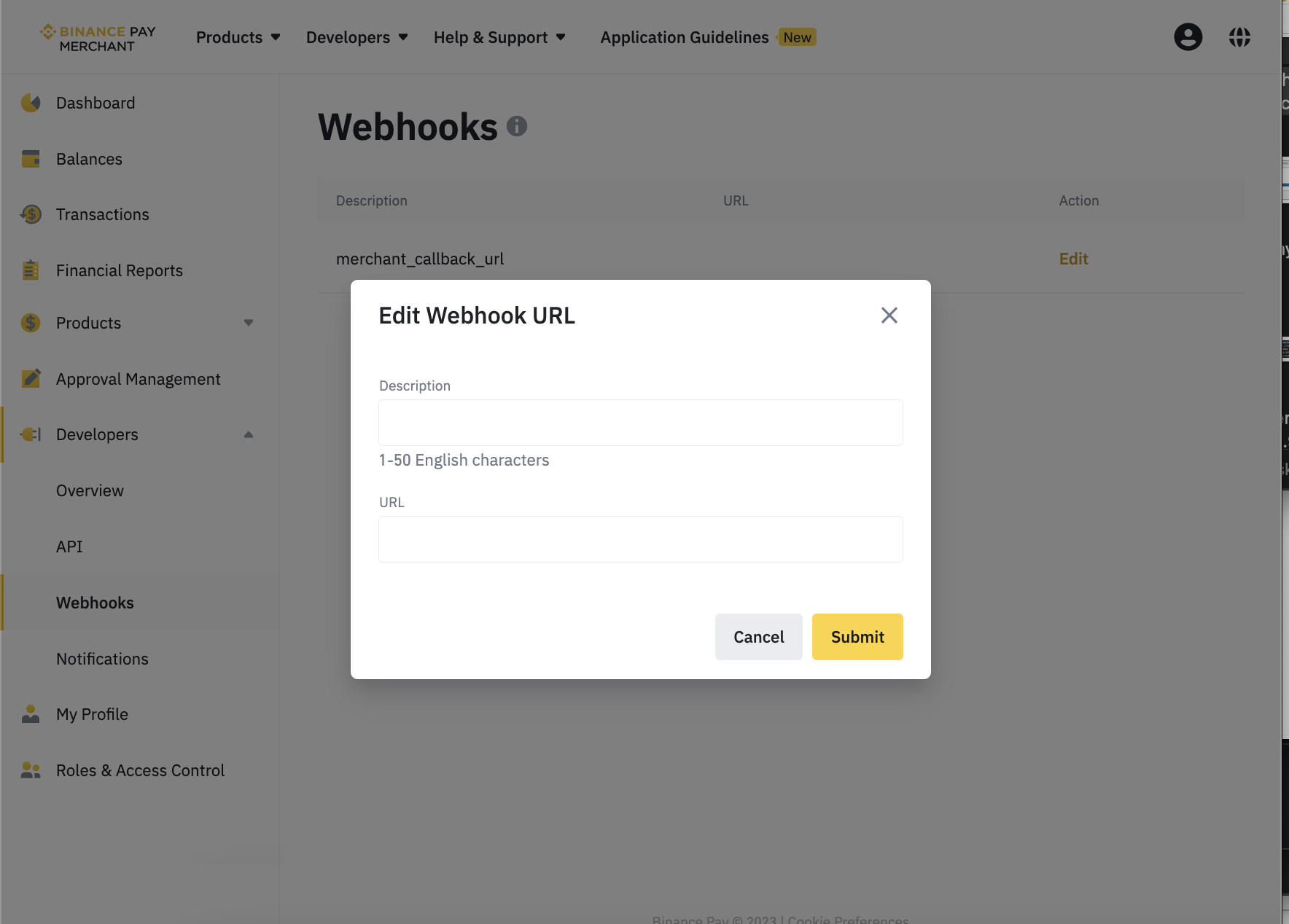
You can also view if the webhook notification has been successfully pushed to your site from the [Notification Log] tab.
#
Webhooks Common RulesFor Security Purpose, Binance will add a signature for webhook notification. Partner needs to verify the signature using the public key issued from Binance Pay.
Please follow the below rules for calling the Webhook Notification from Binance Pay.
Rule | Description |
---|---|
Transfer Mode | Use HTTPS for secure transactions. |
Submit Mode | POST/GET, depends on the API. |
Data Format | The transaction has been paid. |
Char Encoding | Use UTF-8 character encoding. |
Signature Algorithm | RSA, asymmetric cryptographic algorithm |
Signature Requirement | Signature-checking is required for requesting and receiving data |
Logic Judgment | Determine protocol field, service field and transaction status. |
#
Request HeaderAttributes | Type | Required | Description |
---|---|---|---|
BinancePay-Certificate-SN | long | Y | MD5 hash value of public key |
BinancePay-Nonce | string | Y | A random string with 32 bytes, e.g. random asci... |
BinancePay-Timestamp | string | Y | UnixTimestamp in milliseconds |
BinancePay-Signature | string | Y | Signature, generated by Binance Pay |
For full protocol rules specification, please go to Webhook Common Rules.
#
Verify Signatures#
Build the payloadJava
String payload = timestamp + "\n" + nonce + "\n" + body + "\n";
PHP
$payload = $headers['Binancepay-Timestamp'] . "\n" . $headers['Binancepay-Nonce'] . "\n" .$entityBody . "\n";
Note: ‘\n’ is LF, ASCII value is '0x0A'
#
Decode the Signature with Base64Java
byte[] decodedSignature = Base64.getDecoder().decode(signature);
PHP
$decodedSignature = base64_decode ( $headers['Binancepay-Signature'] );
#
Verify the content with public keyJava
// input: pubKeyStr, decodedSignature, payload PEMParser pubParser = new PEMParser(new StringReader(pubKeyStr)) SubjectPublicKeyInfo pubKeyObj = (SubjectPublicKeyInfo) pubParser.readObject(); AsymmetricKeyParameter pubKey = PublicKeyFactory.createKey(pubKeyObj);
byte[] payloadBytes = payload.getBytes(StandardCharsets.UTF_8); RSADigestSigner verifier = new RSADigestSigner(new SHA256Digest()); verifier.init(false, pubKey); verifier.update(payloadBytes, 0, payloadBytes.length); return verifier.verifySignature(decodedSignature);
PHP
openssl_verify($payload, $decodedSignature, $publicKey, OPENSSL_ALGO_SHA256 );
Note: Hash algorithm should use SHA256
#
Query CertificateMerchants can use the “query certificate” API to obtain the public key and Hash Key.
#
End PointPOST /binancepay/openapi/certificates
#
Response ParametersAttributes | Type | Required | Description |
---|---|---|---|
certSerial | string | Y | public key hash value |
certPublic | string | Y | public key |
#
Webhook ExampleMerchants can use the “query certificate” API to obtain the public key and Hash Key.
#
Order NotificationBinance Pay will notify Merchant the final status of the order through webhooks. Orders that are successfully closed will be under bizStatus = "PAY_CLOSED".
#
Payload ExampleYou can either use “merchantTradeNo” or “prepayId” to search for a specific order. Please input at least one of them.
{ "bizType": "PAY", "data": "{\"merchantTradeNo\":\"9825382937292\",\"totalFee\":0.88000000,\"transactTime\":1619508939664,\"currency\":\"USDT\",\"openUserId\":\"1211HS10K81f4273ac031\",\"productType\":\"Food\",\"productName\":\"Ice Cream\",\"tradeType\":\"WEB\",\"transactionId\":\"M_R_282737362839373\"}", "bizId": 29383937493038367292, "bizStatus": "PAY_SUCCESS"}
To ensure that your server has received and accepted the notifications, please acknowledge every notification with a HTTP 200 and SUCCESS response in the body.
#
Response Example{ "returnCode":"SUCCESS", "returnMessage":null}
Orders with no payment activity over 24 hours will be closed automatically, please contact Binance Merchant Operation in order to get the notification.
#
Payout NotificationBinance Pay will notify Merchant of the final status of the batch payout request initiated.
#
Payload Example{ "bizType": "PAYOUT", "data": "{\"batchStatus\":\"SUCCESS\",\"currency\":\"USDT\",\"merchantId\":100100006288,\"requestId\":\"gg8127129\",\"totalAmount\":2.00000000,\"totalNumber\":2}", "bizId": 29383937493038367292, "bizStatus": "SUCCESS"}
To ensure that your server has received and accepted the notifications, please acknowledge every notification with a HTTP 200 and SUCCESS response in the body.
#
Response Example{ "returnCode":"SUCCESS", "returnMessage":null}
#
Refund NotificationBinance Pay will send refund order events with final status when you use the refund open API.
Successful close result for the close refund order request will be notified through this webhook bizStatus is "REFUND_SUCCESS"
or "REFUND_REJECTED"
.
#
Payload Example{ "bizType": "PAY_REFUND", "data":"{\"merchantTradeNo\":\"6177e6ae81ce6f001b4a6233\", \"totalFee\":0.01,\ "transactTime\":1635248421335,\"refundInfo\":{\"orderAmount\":\"0.01000000\",\"duplicateRequest\":\"N\",\"payerOpenId\":\"9aa0a8bb21cf5fbf049aad7db35dc3d3\",\"prepayId\":\"123289163323899904\",\"refundRequestId\":\"68711039982968853\",\"refundedAmount\":\"0.01000000\",\"remainingAttempts\":9,\"refundAmount\":\"0.01000000\"},\"currency\":\"USDT\",\"commission\":0,\"openUserId\":\"b5ec36baaa5ab9a5cfb1c29c2057bd81\",\"productType\":\"LIVE_STREAM\",\"productName\":\"LIVE_STREAM\",\"tradeType\":\"APP\"}", "bizId": 123289163323899904, "bizStatus": "REFUND_SUCCESS"}
To ensure that your server has received and accepted the notifications, please acknowledge every notification with a HTTP 200 and SUCCESS response in the body.
#
Response Example{ "returnCode":"SUCCESS", "returnMessage":null}